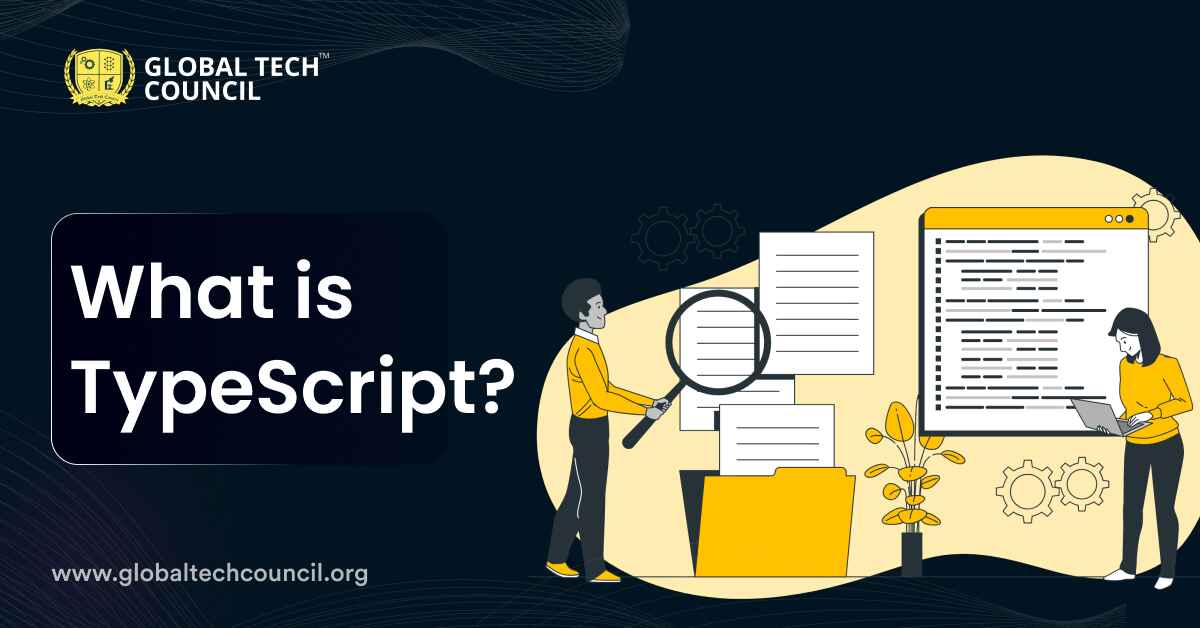
In 2023, TypeScript solidified its position in the programming world by overtaking Java as the third most popular language on GitHub. It marked a significant 37% growth in its user base. This rise proves TypeScript is here to stay.
But what exactly is TypeScript and why is it so popular? Let’s find out!
- Typescript Vs Javascript
- Typescript Interface
- Mapping in Typescript
- React Typescript
- Create React App Typescript
- Typescript Tutorial
- Typescript Types
What is TypeScript?
TypeScript is an open-source, object-oriented, statically typed programming language developed by Microsoft. It is designed as an extension of JavaScript. It adds optional static typing and other features to help manage larger codebases more effectively.
Simply put, TypeScript is a smarter version of JavaScript. It takes the flexibility of JavaScript and enhances it with tools that help catch errors and bugs during development, before the code is even run. This makes TypeScript a great tool for developers who want to ensure their code is error-free and easy to manage, especially in larger projects.
Features of TypeScript
TypeScript is like an upgrade to JavaScript. Here are some features that make it popular among developers:
- Static Typing: You can tell TypeScript exactly what type of data (like a number, string, or a more complex object) you expect in parts of your code. This way, if you accidentally assign the wrong type, TypeScript will warn you right away. This helps prevent bugs that can be hard to track down later.
- Type Inference: TypeScript is smart enough to guess the type of data you’re dealing with in many cases. For instance, if you assign a number to a variable, TypeScript understands that this variable should always be a number without you having to explicitly say so.
- Better Tool Support: With TypeScript, the tools you use to write code can better assist you by offering helpful suggestions, catching errors early, and making it easier to navigate through your code.
- Compatibility with JavaScript: Since TypeScript is built on top of JavaScript, you can use it with all the JavaScript code, libraries, and tools that already exist. You can even convert a JavaScript file to TypeScript just by changing the file extension from .js to .ts and start adding TypeScript features gradually.
- Object-Oriented Features: TypeScript supports features like classes and interfaces, which are ways to organize and structure your code so it’s easier to manage, especially as it grows larger.
- Generics: These allow you to create components that work with any type of data but still keep your code safe and predictable. It’s like creating a tool that can handle different shapes and sizes but still performs its function correctly depending on what you pass to it.
- Union and Intersection Types: TypeScript lets you define variables that might be one type or another (union) or ensure that a variable meets several type criteria all at once (intersection), which is useful for more complex coding scenarios.
- Cross-Platform: You can run TypeScript on any device or browser where JavaScript runs. This means you can use it for websites, server backends, and even mobile apps.
Use Cases of TypeScript
- Developers use TypeScript to make websites. Because it checks your code as you write it, TypeScript helps you catch mistakes early. This means fewer problems when the website is running.
- Large companies with complex websites and apps find TypeScript very helpful. It helps them keep their big projects organized and reduces the number of errors in their code.
- TypeScript works well with well-known tools that help build websites and apps, like Angular, React, and Vue.js. For example, Angular uses TypeScript as its main language because it helps make more reliable applications.
- TypeScript is also used in mobile app development. It helps developers write code that can be used on different types of devices without having to rewrite everything.
- For applications that need to update information immediately, like messaging apps, TypeScript is a good choice because it helps manage the complexity and keeps the app running smoothly.
Examples of Companies Using TypeScript
- Microsoft uses it in many of their products, like the Visual Studio Code editor.
- Google uses TypeScript for Angular, which is a framework for building dynamic web pages.
- Slack and Airbnb use TypeScript because it makes their large codebases easier to handle and helps find errors before the software is even run.
- Asana, a project management tool, uses TypeScript to keep its application organized and efficient.
Coding with TypeScript: Sample Code
Now that you know what TypeScript is and what its features are, let’s have an idea on how to use it. The example below will define an interface for a user, a class that implements this interface, and a function to create a new user and print details about them. This is a typical use case in many applications to ensure object structures are adhered to throughout the application:
// Define an interface for User
interface User {
id: number;
name: string;
age?: number; // The ‘?’ makes the age property optional
}
// Class that implements the User interface
class Employee implements User {
public id: number;
public name: string;
public age?: number;
constructor(id: number, name: string, age?: number) {
this.id = id;
this.name = name;
this.age = age;
}
describe(): string {
return `Employee ${this.id}: ${this.name}, ${this.age ? this.age + ‘ years old’ : ‘age unknown’}.`;
}
}
// Function to create an employee and return description
function createEmployee(id: number, name: string, age?: number): string {
let employee = new Employee(id, name, age);
return employee.describe();
}
// Usage of the function
console.log(createEmployee(1, ‘Alice’, 30));
console.log(createEmployee(2, ‘Bob’));
How to Run TypeScript Code?
To run TypeScript, you first need to install Node.js and TypeScript. Here’s how you can set it up and run the TypeScript code:
- Install Node.js: Download and install it from nodejs.org.
- Install TypeScript: Open your terminal or command prompt and install TypeScript using npm (Node Package Manager):
npm install -g typescript
- Compile TypeScript: Save your TypeScript file (e.g., example.ts) and compile it by running:
tsc example.ts
This command converts your TypeScript file into a JavaScript file (example.js).
- Run the JavaScript file with Node.js:
node example.js
Advantages and Disadvantages of TypeScript
Pros | Cons |
Static Type Checking: Catches bugs early. | Learning Curve: Requires understanding of JavaScript. |
Enhanced Tooling: Advanced IDE features. | Additional Compilation: Transpiles to JavaScript. |
Code Organization: Supports classes, interfaces. | Unsound Type System: Possible runtime errors. |
Popular in Industry: Used by major platforms. | Verbose: More code due to type annotations. |
Gradual Adoption: Easy integration in JS projects. | Overhead: Maintenance of types for smaller projects. |
Conclusion
Through our exploration, it’s clear that TypeScript’s growth is not just a trend but a reflection of its substantial impact on web development. It addresses JavaScript’s limitations and introduces type safety.
Looking ahead, TypeScript’s role in the programming ecosystem is likely to expand. It will continue to support developers in building more reliable and efficient applications. So, if you want to upskill yourself, TypeScript is a must learn language.
When we are talking about upskilling, offers just make the deal better. Check out the latest offers on our globally recognized tech certifications and get ahead of the game!
FAQs
What is TypeScript and why is it popular?
- TypeScript is an open-source, object-oriented, statically typed programming language developed by Microsoft.
- It enhances JavaScript with features like static typing, type inference, and better tool support.
- Its popularity stems from its ability to catch errors early, manage larger codebases effectively, and its compatibility with existing JavaScript code and tools.
What are the key features of TypeScript?
- Static Typing: Helps detect errors during development by specifying data types.
- Type Inference: Automatically infers the data type based on context, reducing the need for explicit type declarations.
- Better Tool Support: Provides advanced IDE features for code navigation, error detection, and refactoring.
- Object-Oriented Features: Supports classes, interfaces, and other object-oriented programming concepts.
- Compatibility with JavaScript: Can be used alongside existing JavaScript code, libraries, and tools.
How is TypeScript used in web development?
- TypeScript helps developers build more reliable and efficient web applications by catching errors early in the development process.
- It’s favored by large companies with complex websites and applications due to its ability to manage large codebases and reduce the number of errors.
- TypeScript integrates well with popular web development frameworks like Angular, React, and Vue.js, further enhancing its utility in web development projects.
Who uses TypeScript and for what purposes?
- Major tech companies like Microsoft, Google, Slack, and Airbnb use TypeScript in their projects for improved code organization and error prevention.
- It’s commonly used in various domains such as web development, mobile app development, and enterprise software development.
- TypeScript is preferred for projects that require reliability, scalability, and maintainability, making it suitable for both small and large-scale applications.
Leave a Reply